woob library¶
Woob (Web Outside of Browsers) is a library which provides a Python standardized API and data models to access websites.
Abstract¶
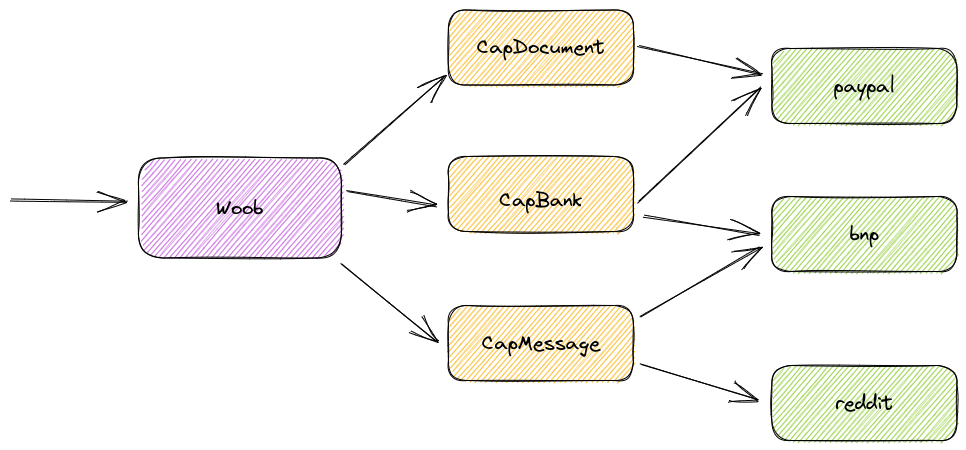
There are three main concepts:
Capabilities: This is a standardized interface to access a specific kind of website. It provides an unified API and standard datamodels;
Modules: A module is dedicated to a specific website. It can implements several capabilities (for example paypal module may implement
CapBank
to get bank informations,CapBankTransfer
to initiate a transfer,CapProfile
to get information about the customer, andCapDocument
to get documents);Backends: You can load a module several times, with different configurations. For example, if you have two PayPal accounts, you can create two backends of the same module with different credentials.
The main Woob
class let configure new backends and do
aggregated calls to every backends loaded with a specific capability.
For example, once several backends are loaded, you can call
iter_accounts()
and you’ll get
accounts in the same Account
data model
for all backends implementing CapBank
:
>>> from woob.core import Woob
>>> from woob.capabilities.bank import CapBank
>>> w = Woob()
>>> w.load_backends(CapBank)
{'societegenerale': <Backend 'societegenerale'>,
'creditmutuel': <Backend 'creditmutuel'>}
>>> accounts = list(w.iter_accounts())
>>> print(accounts)
[<Account id='7418529638527412' label=u'Compte de ch\xe8ques'>,
<Account id='9876543216549871' label=u'Livret A'>,
<Account id='123456789123456789123EUR' label=u'C/C Eurocompte Confort M Roger Philibert'>]
>>> accounts[0].balance
Decimal('87.32')
Documentation¶
Examples¶
Video search¶
>>> from woob.core import Woob
>>> from woob.capabilities.bank import CapVideo
>>> w = Woob()
>>> w.load_backend(CapVideo)
{'vimeo': <Backend 'vimeo'>,
'youtube': <Backend 'youtube'>}
>>> for video in w.search_videos('pink floyd'):
... print(f'{video.id}@{video.backend}: {video.title}')
...
Hh1Xyvu68P0@youtube: P̲ink F̲lo̲yd - T̲h̲e̲ D̲ark S̲ide̲ Of T̲h̲e̲ Mo̲o̲n (Full Album) 1973
IXdNnw99-Ic@youtube: Pink Floyd - Wish You Were Here
cuKVhVb8VRw@youtube: The D̲ark S̲ide of the Mo̲o̲n (Full Album) 1973
_FrOQC-zEog@youtube: Pink Floyd - Comfortably numb
191099965@vimeo: Pink Floyd - Echoes
184017902@vimeo: Pink Floyd - Dogs
94573195@vimeo: Pink Panther Floyd
31536402@vimeo: PINK FLOYD - PULSE
Banking aggregation¶
>>> from woob.core import Woob
>>> w = Woob()
>>> w.load_backend('societegenerale', 'soge',
... config={'login': '1234', 'password': '5678'})
<Backend 'soge'>
>>> w.load_backend('creditmutuel', 'cm',
... config={'login': '1234', 'password': '5678'})
<Backend 'cm'>
>>> for account in w.iter_accounts():
... print(f'{account.id}@{account.backend} {account.label:>40} {account.balance} {account.currency_text}')
1234567789@soge Compte chèque 1528.39 €
DL00012345@cm COMPTE CHEQUES 1 ROGER PHILIBERT 100.00 €
DD99874634@cm PRET PRIMO ACCEDANT - ACHAT D'ANCIEN SEUL -9555.52 €
>>> account = w['cm'].get_account('DD99874634')
>>> account
<Loan id='DD99874634' label="PRET PRIMO ACCEDANT - ACHAT D'ANCIEN SEUL">
>>> account.subscription_date
date(2022, 6, 2)
>>> account.total_amount
Decimal('10000.0')
>>> account.next_payment_amount
Decimal('59.43')
>>> account.next_payment_date
date(2023, 3, 5)
See woob.capabilities.bank.base
.